Simulation of
advection-diffusion
In this assignment you should use OpenGL to perform a simple 2D-animation
of a particle system, simulating advection and diffusion.
Here is one frame of an animation:
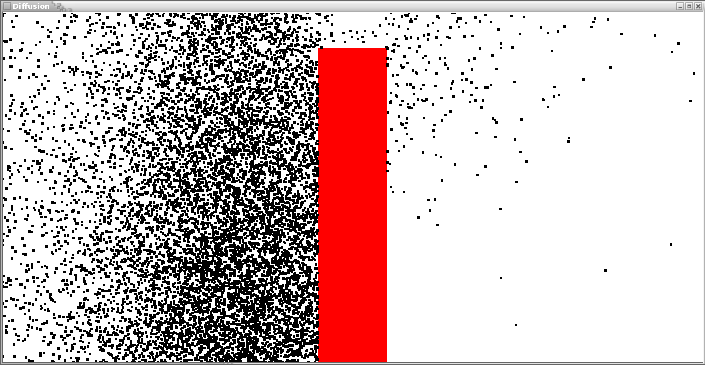
You see a rectangle filled with 10 000 particles. The particles may
not leak out from the rectangle nor can they penetrate the red barrier.
The particles will move through simulated diffusion and advection. The
simulation should continue until you stop it.
Diffusion is a small-scale phenomenon where particles, e.g. atoms or
molecules, move by thermal energy. Advection, on the other hand,
occurs on a large-scale,
think of water flowing in a river or air transported by the wind.
Diffusion and advection can be taking place at the same time. For much
more background (not needed for this lab) see the Wikipedia article
about diffusion and advection.
Here are the requirements:
- The rectangle is defined by 0 <= x <= 2, 0 <= y <= 1,
and the barrier has corners in (0.9, 0), (0.9, 0.9), (1.1, 0.9)
and (1.1, 0).
- In each time step you should update all the positions of the
particles and then update the display. You can consider one particle at
a time when recomputing the position, you do not have to consider interactions
between particles.
- To simulate diffusion (Brownian motion) we make the following
construction. Assume (x, y) is the present location of one of the
particles. The new position of the particle is (x + scale · rand_x, y +
scale · rand_y) where rand_x, rand_y are two uniformly distributed
random numbers in the interval (-0.005, 0.005). You should of course
use different random numbers for all particles (and for x and y).
scale is a scale factor initially set to one. By pressing the letter s
on the keyboard, scale can be decreased, 0.01 per keypress. Pressing S
will increase scale by 0.01. The scale factor may not become negative
(but zero is OK).
Using the scale factor one can simulate a change in temperature. In an
ideal gas with equal mass of the partices the temperature is
proportional to the average speed squared.
- To simulate advection we define the new position to be (x + scale
· rand_x + a_x, y + scale · rand_y + a_y). a_x and a_y are initially
zero. Pressing x on the keyboard should decrease a_x by 0.0005,
pressing X should increase a_x by 0.0005. Analogously for pressing y
and Y.
Pressing y one can simulate gravity as well.
- If the new position of a particle is outside the rectangle or
inside
the barrier, move it to an appropriate position on the boundary of
rectangle/barrier. You do not
have to simulate bouncing.
- If one presses the letter r (for reset), a_x, a_y and scale
should be reset to their initial values (the animation should
continue). Pressing q should terminate the execution of the program.
- The initial position of the particles should be decided by one
click of the mouse. The position of the mouse click should be the
center of a square having side 0.05. Randomly generate positions for
the 10 000 particles inside this square. Particles, falling outside the
rectangle or inside the barrier, should be repositioned as described
above.
- There should be a menu with two alternatives, Reset (see above)
and Quit.
Your program does not have to be more fancy than my example code,
but you may make a more fancy version provided it fulfills the
requirements above.
Hint:
read the Linux manual page for rand
(to make random numbers in C). Note that rand in my description above is not the same as the rand-function
in the C-library.
